Mastering Go's Context: From Basics to Best Practices
Go's context
package is a powerful tool for managing cancellation, deadlines, and request-scoped values across API boundaries and between processes. While essential for writing robust and efficient Go code, it can be challenging to use correctly. This comprehensive guide will walk you through everything you need to know about Go's context, from its basic concepts to advanced usage patterns.
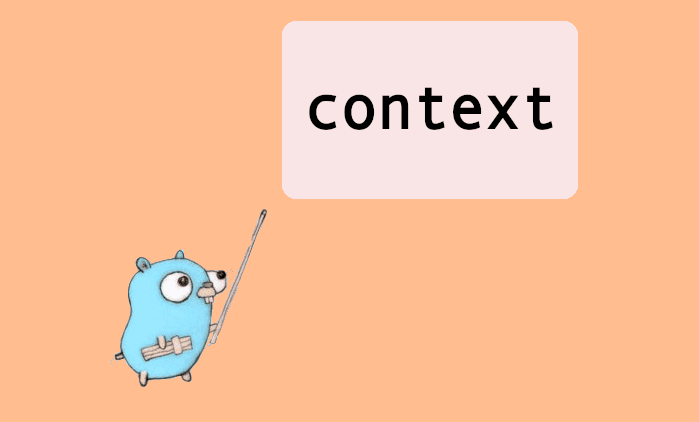
Introduction to Go Context
Go's context
package is a fundamental tool in the Go programming language, designed to solve several critical problems in concurrent and networked applications. Introduced in Go 1.7, the context package provides a standardized way to carry deadlines, cancellation signals, and other request-scoped values across API boundaries and between processes.
The primary purposes of the context package are:
-
Cancellation: Allowing long-running operations to be canceled when they're no longer needed, preventing unnecessary work and resource consumption.
-
Deadlines and Timeouts: Setting time limits on operations to ensure they don't run indefinitely, which is crucial for maintaining responsive systems.
-
Request-Scoped Data: Carrying request-specific information (like user IDs or authentication tokens) through layers of an application without modifying function signatures.
Here's a simple example to illustrate the basic usage of context:
package main
import (
"context"
"fmt"
"time"
)
func main() {
// Create a context with a timeout of 2 seconds
ctx, cancel := context.WithTimeout(context.Background(), 2*time.Second)
defer cancel() // Ensure resources are released
go worker(ctx)
select {
case <-ctx.Done():
fmt.Println("Main: worker completed or timed out")
}
}
func worker(ctx context.Context) {
for {
select {
case <-ctx.Done():
fmt.Println("Worker: I'm done")
return
default:
fmt.Println("Worker: Doing work...")
time.Sleep(500 * time.Millisecond)
}
}
}
In this example, we create a context with a 2-second timeout. The worker function continuously checks if the context is done, allowing it to gracefully stop when the timeout occurs or when the context is explicitly canceled.
Core Components of Context
The Go context package consists of several key components that work together to provide its functionality.
Context Interface
The heart of the package is the Context
interface, which defines four methods:
type Context interface {
Deadline() (deadline time.Time, ok bool)
Done() <-chan struct{}
Err() error
Value(key interface{}) interface{}
}
Deadline()
: Returns the time when the context will be canceled, if set.Done()
: Returns a channel that's closed when the context is canceled.Err()
: Returns the error indicating why the context was canceled.Value()
: Returns the value associated with a key, or nil if no value is associated.
Background() and TODO() Functions
These functions return empty contexts, which serve as the root of a context tree:
func Background() Context
func TODO() Context
Background()
: Returns a non-nil, empty Context. It's typically used as the root context for incoming requests in a server.TODO()
: Similar to Background(), but should be used when it's unclear which Context to use or it's not yet available.
Example usage:
ctx := context.Background()
// Use ctx as the root context
WithCancel(), WithDeadline(), WithTimeout() Functions
These functions create derived contexts with cancellation capabilities:
func WithCancel(parent Context) (ctx Context, cancel CancelFunc)
func WithDeadline(parent Context, d time.Time) (Context, CancelFunc)
func WithTimeout(parent Context, timeout time.Duration) (Context, CancelFunc)
WithCancel()
: Creates a new context that can be manually canceled.WithDeadline()
: Creates a context that will be canceled at a specific time.WithTimeout()
: Creates a context that will be canceled after a duration.
Example usage:
ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second)
defer cancel() // Always call cancel to release resources
// Use ctx for operations that should be canceled after 5 seconds
WithValue() Function
This function creates a derived context with a key-value pair:
func WithValue(parent Context, key, val interface{}) Context
It's used to pass request-scoped values across API boundaries.
Example usage:
ctx := context.WithValue(context.Background(), "userID", "user-123")
// Later, retrieve the value
if userID, ok := ctx.Value("userID").(string); ok {
fmt.Println("User ID:", userID)
}
In the next sections, we'll explore how to use these components in practical scenarios and discuss best practices for leveraging the full power of Go's context package.
Key Use Cases for Context
The context package in Go is versatile and finds application in various scenarios. Let's explore three primary use cases that demonstrate the power and flexibility of context.
Cancellation
One of the most common use cases for context is managing cancellation of operations. This is particularly useful for long-running processes or when you need to stop work across multiple goroutines.
Example:
func main() {
ctx, cancel := context.WithCancel(context.Background())
defer cancel()
go worker(ctx, "Worker 1")
go worker(ctx, "Worker 2")
time.Sleep(3 * time.Second)
cancel() // Cancel all workers
time.Sleep(1 * time.Second) // Give workers time to stop
}
func worker(ctx context.Context, name string) {
for {
select {
case <-ctx.Done():
fmt.Printf("%s: Stopped\n", name)
return
default:
fmt.Printf("%s: Working\n", name)
time.Sleep(1 * time.Second)
}
}
}
In this example, multiple workers can be canceled simultaneously by calling the cancel function, demonstrating how context can manage cancellation across goroutines.
Deadlines and Timeouts
Context allows you to set deadlines or timeouts for operations, which is crucial for preventing long-running operations from consuming resources indefinitely.
Example:
func main() {
ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second)
defer cancel()
result, err := fetchData(ctx)
if err != nil {
if err == context.DeadlineExceeded {
fmt.Println("Operation timed out")
} else {
fmt.Println("Error:", err)
}
return
}
fmt.Println("Result:", result)
}
func fetchData(ctx context.Context) (string, error) {
select {
case <-time.After(6 * time.Second): // Simulating a slow operation
return "Data fetched", nil
case <-ctx.Done():
return "", ctx.Err()
}
}
This example demonstrates how to use context to set a timeout for an operation. If the operation takes longer than the specified timeout, it's canceled and an error is returned.
Passing Request-Scoped Values
Context allows you to pass request-scoped values across API boundaries without having to modify function signatures.
Example:
type key int
const userIDKey key = iota
func main() {
ctx := context.WithValue(context.Background(), userIDKey, "user-123")
processRequest(ctx)
}
func processRequest(ctx context.Context) {
userID, ok := ctx.Value(userIDKey).(string)
if !ok {
fmt.Println("No user ID found in context")
return
}
fmt.Println("Processing request for user:", userID)
// Perform operations using the user ID
}
In this example, we're passing a user ID through the context. This is particularly useful in web applications where you might want to pass authentication information or request IDs through multiple layers of your application.
In the next section, we'll discuss best practices for using context to ensure you're getting the most out of this powerful package while avoiding common pitfalls.
Best Practices for Using Context
While the context package is powerful and flexible, it's important to use it correctly to avoid common pitfalls and ensure your code remains readable and maintainable. Let's explore some best practices for using context effectively in your Go programs.
Passing Context as the First Parameter
A widely accepted convention in Go is to pass the context as the first parameter of a function. This practice improves code readability and makes it clear that the function supports cancellation or has a deadline.
Good practice:
func ProcessData(ctx context.Context, data []byte) error {
// Use ctx here
}
Not recommended:
func ProcessData(data []byte, ctx context.Context) error {
// Context should be the first parameter
}
By consistently following this pattern, you make your code more idiomatic and easier for other Go developers to understand and use.
Proper Error Handling with Context
When using context for cancellation or timeouts, it's crucial to handle errors properly. Always check for context cancellation and return appropriate errors.
Example of proper error handling:
func DoOperation(ctx context.Context) (Result, error) {
select {
case <-ctx.Done():
return Result{}, ctx.Err() // Could be Canceled or DeadlineExceeded
case result := <-doSomethingLong():
return result, nil
}
}
func main() {
ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second)
defer cancel()
result, err := DoOperation(ctx)
if err != nil {
switch err {
case context.Canceled:
fmt.Println("Operation was canceled")
case context.DeadlineExceeded:
fmt.Println("Operation timed out")
default:
fmt.Println("Operation failed:", err)
}
return
}
// Use result
}
Using Context Values Appropriately
While context.Value is useful for passing request-scoped data, it should be used prudently. Overuse can lead to unclear dependencies and make code harder to maintain.
Best practices for using context.Value:
- Use it for request-scoped data that transits process or API boundaries, not for passing optional parameters to functions.
- The key should be a custom type to avoid collisions.
- The value should be immutable and safe for simultaneous use by multiple goroutines.
Example of appropriate use:
type contextKey string
const (
userIDKey contextKey = "userID"
requestIDKey contextKey = "requestID"
)
func ProcessRequest(ctx context.Context) {
userID, ok := ctx.Value(userIDKey).(string)
if !ok {
// Handle missing user ID
}
requestID, ok := ctx.Value(requestIDKey).(string)
if !ok {
// Handle missing request ID
}
// Use userID and requestID in processing
}
func main() {
ctx := context.Background()
ctx = context.WithValue(ctx, userIDKey, "user-123")
ctx = context.WithValue(ctx, requestIDKey, "req-456")
ProcessRequest(ctx)
}
In this example, we're using context.Value to pass request-specific information (user ID and request ID) that might be needed across multiple layers of an application.
In the next section, we'll discuss common pitfalls and misconceptions to avoid when working with the context package.
Common Pitfalls and Misconceptions
While the context package is a powerful tool in Go, it's easy to misuse if you're not familiar with its intricacies. Let's explore some common pitfalls and misconceptions developers often encounter when working with context.
Storing Contexts in Structs
One common mistake is storing a context in a struct. This is generally considered bad practice because a context is designed to be canceled or to expire, and storing it in a struct can lead to unexpected behavior.
Incorrect usage:
type BadService struct {
ctx context.Context
// other fields
}
func NewBadService(ctx context.Context) *BadService {
return &BadService{ctx: ctx}
}
Instead, pass the context as a parameter to methods that need it:
type GoodService struct {
// other fields
}
func (s *GoodService) DoSomething(ctx context.Context) error {
// Use ctx here
}
By passing the context as a parameter, you ensure that each method call can have its own context, which can be canceled or have a deadline without affecting other operations.
Ignoring Context Cancellation
Another common pitfall is ignoring context cancellation. This can lead to goroutine leaks and unnecessary work being performed.
Incorrect usage:
func longRunningOperation(ctx context.Context) {
for {
// Do some work
time.Sleep(time.Second)
}
}
Correct usage:
func longRunningOperation(ctx context.Context) {
for {
select {
case <-ctx.Done():
// Clean up and return
return
default:
// Do some work
time.Sleep(time.Second)
}
}
}
Always check for context cancellation in long-running operations to ensure your goroutines can be properly terminated.
Misusing Context Values
While context.Value
is useful for passing request-scoped data, it's often misused for passing function parameters or configuration options.
Incorrect usage:
func ProcessData(ctx context.Context) {
dbConn := ctx.Value("dbConnection").(Database)
// Use dbConn
}
func main() {
ctx := context.Background()
ctx = context.WithValue(ctx, "dbConnection", myDatabase)
ProcessData(ctx)
}
Instead, pass important dependencies explicitly:
func ProcessData(ctx context.Context, dbConn Database) {
// Use dbConn
}
func main() {
ctx := context.Background()
ProcessData(ctx, myDatabase)
}
Use context.Value
for request-scoped data that isn't critical to the function's operation, such as trace IDs or user information. For essential dependencies, pass them as explicit parameters.
Forgetting to Cancel Contexts
When creating a new context with WithCancel
, WithDeadline
, or WithTimeout
, it's crucial to call the cancel function, even if the context has already expired.
Incorrect usage:
ctx, cancel := context.WithTimeout(parentCtx, 5*time.Second)
// Use ctx
// Forget to call cancel()
Correct usage:
ctx, cancel := context.WithTimeout(parentCtx, 5*time.Second)
defer cancel() // Always call cancel to release resources
// Use ctx
Failing to cancel contexts can lead to resource leaks, as the parent context will retain references to the child until the parent is canceled or the program exits.
In the next section, we'll explore some advanced usage patterns for the context package, building on the knowledge we've gained so far.
Advanced Context Usage
Now that we've covered the basics and common pitfalls, let's explore some advanced techniques for using context in Go. These patterns will help you leverage the full power of the context package in more complex scenarios.
Chaining Contexts
You can create a chain of contexts, each adding its own values or cancellation conditions. This is useful when you want to combine multiple behaviors or pass along context values while adding new ones.
Example of context chaining:
func main() {
// Root context
ctx := context.Background()
// Add a timeout
ctx, cancel := context.WithTimeout(ctx, 10*time.Second)
defer cancel()
// Add a value
ctx = context.WithValue(ctx, "requestID", "12345")
// Create a cancelable child context
childCtx, childCancel := context.WithCancel(ctx)
defer childCancel()
go doSomething(childCtx)
// Wait for a while, then cancel the child context
time.Sleep(5 * time.Second)
childCancel()
}
func doSomething(ctx context.Context) {
for {
select {
case <-ctx.Done():
fmt.Println("Operation canceled, cleaning up...")
return
default:
rid, _ := ctx.Value("requestID").(string)
fmt.Printf("Doing something with request ID: %s\n", rid)
time.Sleep(1 * time.Second)
}
}
}
In this example, we chain multiple contexts together, adding a timeout, a value, and a cancellation mechanism. The resulting context inherits all these properties.
Context in HTTP Servers
Context is particularly useful in HTTP servers for managing request-scoped data and timeouts. Go's http.Request
type includes a Context
method that returns a context for each request.
Example of using context in an HTTP server:
func main() {
http.HandleFunc("/", handleRequest)
http.ListenAndServe(":8080", nil)
}
func handleRequest(w http.ResponseWriter, r *http.Request) {
// Get the request's context
ctx := r.Context()
// Add a timeout to the request context
ctx, cancel := context.WithTimeout(ctx, 5*time.Second)
defer cancel()
// Add a value to the context
ctx = context.WithValue(ctx, "userID", r.Header.Get("User-ID"))
// Use the context in a goroutine
resultChan := make(chan string, 1)
go doSlowOperation(ctx, resultChan)
select {
case result := <-resultChan:
fmt.Fprintf(w, "Result: %s", result)
case <-ctx.Done():
http.Error(w, "Request timed out", http.StatusRequestTimeout)
}
}
func doSlowOperation(ctx context.Context, resultChan chan<- string) {
userID, _ := ctx.Value("userID").(string)
select {
case <-time.After(6 * time.Second):
resultChan <- fmt.Sprintf("Operation completed for user %s", userID)
case <-ctx.Done():
fmt.Println("Operation canceled")
}
}
This example demonstrates how to use context in an HTTP server to manage request timeouts and pass request-scoped data.
Working with Goroutines and Context
When working with goroutines, context can be used to coordinate cancellation and manage the lifecycle of background tasks.
Example of managing multiple goroutines with context:
func main() {
ctx, cancel := context.WithCancel(context.Background())
defer cancel()
// Start multiple workers
for i := 0; i < 3; i++ {
go worker(ctx, i)
}
// Simulate some work
time.Sleep(5 * time.Second)
// Cancel all workers
cancel()
// Wait for workers to finish
time.Sleep(1 * time.Second)
}
func worker(ctx context.Context, id int) {
for {
select {
case <-ctx.Done():
fmt.Printf("Worker %d shutting down\n", id)
return
default:
fmt.Printf("Worker %d doing work\n", id)
time.Sleep(1 * time.Second)
}
}
}
This example shows how to use a single context to manage multiple goroutines, allowing for coordinated shutdown of all workers.
Conclusion
Let's recap the key points we've covered and reflect on the importance of mastering context in Go programming.
-
Core Components: We explored the fundamental elements of the context package, including the Context interface, Background() and TODO() functions, and various With* functions for creating derived contexts.
-
Key Use Cases: We examined the primary applications of context, such as managing cancellation, setting deadlines and timeouts, and passing request-scoped values across API boundaries.
-
Best Practices: We discussed essential best practices, including passing context as the first parameter, proper error handling, and appropriate use of context values.
-
Common Pitfalls: We highlighted frequent mistakes to avoid, such as storing contexts in structs, ignoring context cancellation, and misusing context values.
-
Advanced Usage: We delved into more complex patterns, including chaining contexts, utilizing context in HTTP servers, and coordinating goroutines with context.
As you continue your journey in Go programming, remember that mastering context is not just about knowing its API, but about understanding its design philosophy and applying it wisely in your applications. With practice and careful consideration, you'll find that the context package becomes an indispensable part of your Go programming toolkit, enabling you to tackle complex concurrency challenges with confidence and elegance.
Keep exploring, keep practicing, and most importantly, keep the context in mind as you build your Go applications. Happy coding!