Understanding Go's defer: Usage, Evaluation, and Error Handling
Go's defer
keyword schedules function calls to run just before the current function returns. It's primarily used for resource management and cleanup. While powerful, defer
has specific behaviors that can cause issues if misunderstood.
This article covers:
We'll examine each topic with code examples to show proper usage and potential pitfalls.
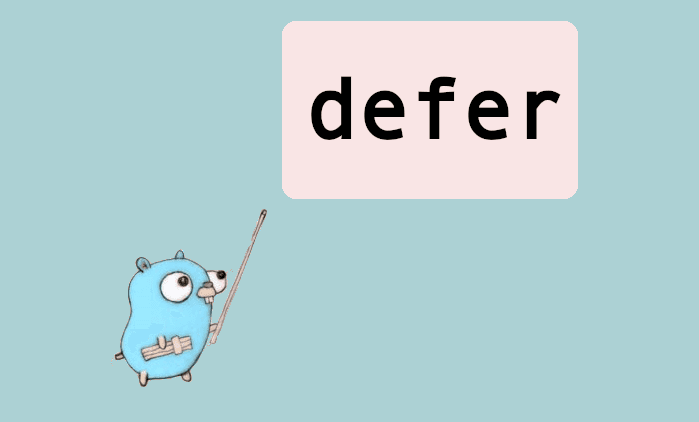
Common Use Cases for Defer
Defer ensures that certain operations happen reliably, regardless of how a function exits. It's particularly useful for:
- Closing files
- Unlocking mutexes
- Cleaning up database connections
Let's explore these use cases with practical examples.
Managing File Resources
One of the most common uses of defer is managing file operations. When you open a file, it's crucial to ensure that the file is closed once you're done to prevent resource leaks. Here's an expanded example:
package main
import (
"fmt"
"log"
"os"
)
func readFile(filename string) {
file, err := os.Open(filename)
if err != nil {
log.Fatalf("Failed to open file: %v", err)
}
defer file.Close() // Ensures that the file is closed when the function exits
// Read file contents (for example, using a buffer)
buffer := make([]byte, 100)
_, err = file.Read(buffer)
if err != nil {
log.Fatalf("Failed to read file: %v", err)
}
fmt.Println("File contents:", string(buffer))
}
In this example, defer file.Close()
guarantees that Close()
is called regardless of whether reading succeeds or fails, maintaining the integrity of resource management.
Locking and Unlocking
For concurrency control, defer is used with mutexes to ensure that locks are properly released, preventing deadlocks:
package main
import (
"sync"
)
var mu sync.Mutex
func criticalSection() {
mu.Lock()
defer mu.Unlock() // Automatically unlocks at the end of the function
// Critical section code
}
By deferring mu.Unlock()
, you ensure that the mutex is unlocked even if the code within the critical section might panic or return early.
Closing Network Connections
When working with network connections, it's important to ensure they are closed after use. Defer can streamline this:
package main
import (
"log"
"net"
)
func connect() {
conn, err := net.Dial("tcp", "example.com:80")
if err != nil {
log.Fatalf("Connection failed: %v", err)
}
defer conn.Close()
// Perform network operations
}
When Defer Arguments are Evaluated
A common misconception about defer in Go is when the arguments to a deferred function call are evaluated. It's crucial to understand that the arguments are evaluated immediately when the defer statement is executed, not when the surrounding function returns. This behavior can lead to unexpected results if not properly understood.
Immediate Evaluation
Let's start with a simple example to illustrate this concept:
package main
import "fmt"
func main() {
x := 1
defer fmt.Println("x:", x)
x++
fmt.Println("x in main:", x)
}
Output:
x in main: 2
x: 1
In this example, the value of x
printed by the deferred call is 1, not 2. This is because the argument to fmt.Println
is evaluated when defer is called, capturing the value of x
at that moment.
Function Calls as Arguments
The same principle applies when using function calls as arguments to a deferred function:
package main
import "fmt"
func getValue() int {
return 1
}
func main() {
defer fmt.Println("Value:", getValue())
fmt.Println("Main function")
}
Output:
Main function
Value: 1
Here, getValue()
is called immediately when the defer statement is executed, not when the main function returns.
Solving the Evaluation Issue
To work around this behavior, there are two common approaches:
- Use a function literal (closure):
func main() {
x := 1
defer func() {
fmt.Println("x:", x)
}()
x++
fmt.Println("x in main:", x)
}
Output:
x in main: 2
x: 2
By using a closure, we capture the variable x
itself, not its value at defer time. This allows us to print the final value of x
.
- Pass a pointer:
func main() {
x := 1
defer func(px *int) {
fmt.Println("x:", *px)
}(&x)
x++
fmt.Println("x in main:", x)
}
Output:
x in main: 2
x: 2
By passing a pointer to x
, we ensure that the deferred function will access the current value of x
when it's called.
Practical Example: Timing Function Execution
Understanding this behavior can be useful in scenarios like timing function execution:
package main
import (
"fmt"
"time"
)
func timeTrack(start time.Time, name string) {
elapsed := time.Since(start)
fmt.Printf("%s took %s\n", name, elapsed)
}
func longRunningFunction() {
defer timeTrack(time.Now(), "longRunningFunction")
// Simulate work
time.Sleep(2 * time.Second)
}
func main() {
longRunningFunction()
}
Output:
longRunningFunction took 2s
In this example, time.Now()
is evaluated immediately when defer is called, correctly capturing the start time of the function.
Handling Errors in Defer
Error handling is a crucial aspect of Go programming, and it becomes even more important when working with deferred functions. Proper error handling in deferred calls can prevent silent failures and ensure that your program behaves as expected, even in error scenarios.
The Importance of Error Handling in Defer
When using defer
, it's easy to overlook error handling, especially because deferred functions are often used for cleanup operations that we assume will always succeed. However, these operations can fail, and ignoring their errors can lead to subtle bugs or resource leaks.
Common Pitfalls
Let's look at a common mistake:
func processFile(filename string) error {
f, err := os.Open(filename)
if err != nil {
return err
}
defer f.Close() // This can potentially return an error!
// Process file...
return nil
}
In this example, we're ignoring any potential error from f.Close()
. If closing the file fails, we won't know about it.
Best Practices for Error Handling in Defer
- Explicitly handle or log errors:
func processFile(filename string) (err error) {
f, err := os.Open(filename)
if err != nil {
return err
}
defer func() {
if cerr := f.Close(); cerr != nil {
if err == nil {
err = cerr
} else {
log.Printf("error closing file: %v", cerr)
}
}
}()
// Process file...
return nil
}
In this improved version, we're capturing any error from f.Close()
. If there was no previous error, we return the close error. Otherwise, we log it.
- Use named return values:
Named return values allow you to modify the returned error in the deferred function:
func writeToFile(filename string, data []byte) (err error) {
f, err := os.Create(filename)
if err != nil {
return err
}
defer func() {
if cerr := f.Close(); cerr != nil && err == nil {
err = cerr
}
}()
_, err = f.Write(data)
return // implicit return of named 'err' variable
}
- Explicitly ignore errors when appropriate:
If you've determined that an error can be safely ignored, use the blank identifier to make it clear this is intentional:
defer func() {
_ = f.Close() // Error explicitly ignored
}()
Handling Multiple Deferred Calls
When you have multiple deferred calls, remember that they execute in LIFO (Last In, First Out) order. This can be important for error handling:
func multipleDefers() (err error) {
r1, err := openResource1()
if err != nil {
return err
}
defer func() {
if cerr := r1.Close(); cerr != nil && err == nil {
err = cerr
}
}()
r2, err := openResource2()
if err != nil {
return err
}
defer func() {
if cerr := r2.Close(); cerr != nil && err == nil {
err = cerr
}
}()
// Use r1 and r2...
return nil
}
In this case, r2
will be closed before r1
, which might be important depending on your resources.
Conclusion
Go's defer
is a key feature for resource management and code organization.
- Use
defer
for consistent resource cleanup, such as closing files and network connections, and unlocking mutexes. - Be aware that defer arguments are evaluated immediately, not when the function returns.
- Handle errors in deferred calls explicitly to prevent silent failures and potential resource leaks.
- Use named return values and closures when needed to manage complex defer scenarios.
Correct use of defer
leads to cleaner, more reliable Go code. Practice these concepts in your projects to fully grasp defer
's capabilities and avoid common mistakes.